Проблема со Split в C#
Сам код:
Код:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
public class testov :Form
{
String result = "";
private Button button1;
private RichTextBox richTextBox1;
private RichTextBox richTextBox2;
string[] myarr = { };
public testov() {
this.Text = "programname";
this.Size = new Size(600, 500);
//buttons
button1 = new Button();
button1.Location = new Point(39, 309);
button1.Size = new Size(154, 53);
button1.TabIndex = 0;
button1.Text = "OK";
button1.UseVisualStyleBackColor = true;
button1.Click += new EventHandler(on_click);
this.Controls.Add(button1);
//textboxes
richTextBox1 = new RichTextBox();
richTextBox1.Location = new Point(14, 14);
richTextBox1.Size = new Size(479, 130);
richTextBox1.TabIndex = 1;
richTextBox1.Text = "";
richTextBox2 = new RichTextBox();
richTextBox2.Location = new Point(14, 173);
richTextBox2.Size = new Size(479, 114);
richTextBox2.TabIndex = 1;
richTextBox2.Text = result;
this.Controls.Add(richTextBox1);
this.Controls.Add(richTextBox2);
}
//function
void on_click(object sender, EventArgs e){
myarr = richTextBox1.Text.Split("<SP>"); //тут пишет ошибку
for (int i = 0; i < myarr.Length;i++){
result+=myarr[i];
}
richTextBox2.Text += result;
}
static void Main()
{
Application.Run(new testov());
}
}
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
public class testov :Form
{
String result = "";
private Button button1;
private RichTextBox richTextBox1;
private RichTextBox richTextBox2;
string[] myarr = { };
public testov() {
this.Text = "programname";
this.Size = new Size(600, 500);
//buttons
button1 = new Button();
button1.Location = new Point(39, 309);
button1.Size = new Size(154, 53);
button1.TabIndex = 0;
button1.Text = "OK";
button1.UseVisualStyleBackColor = true;
button1.Click += new EventHandler(on_click);
this.Controls.Add(button1);
//textboxes
richTextBox1 = new RichTextBox();
richTextBox1.Location = new Point(14, 14);
richTextBox1.Size = new Size(479, 130);
richTextBox1.TabIndex = 1;
richTextBox1.Text = "";
richTextBox2 = new RichTextBox();
richTextBox2.Location = new Point(14, 173);
richTextBox2.Size = new Size(479, 114);
richTextBox2.TabIndex = 1;
richTextBox2.Text = result;
this.Controls.Add(richTextBox1);
this.Controls.Add(richTextBox2);
}
//function
void on_click(object sender, EventArgs e){
myarr = richTextBox1.Text.Split("<SP>"); //тут пишет ошибку
for (int i = 0; i < myarr.Length;i++){
result+=myarr[i];
}
richTextBox2.Text += result;
}
static void Main()
{
Application.Run(new testov());
}
}
http://csharp.net-informations.com/string/csharp_string_tutorial.htm
rogal.
А что должна программа делать?
Цитата: UserNet2008
Типа этого, только весь <SP> сепаратор...
Нашёл по ссылке выше пример со сплитом, переделал метод нажатия на кнопку, но типы что-то не хотят преобразовываться:
Код:
void on_click(object sender, EventArgs e){
string[] myarr = null;
char[] splitchar = { "<SP>" }; //ошибка - неявное преобразование
string sep = (string)splitchar; //ошибка - преобразование типов невозможно
myarr = richTextBox1.Text.Split(sep); //ошибка - метод имеет несколько недопустимых аргументов
for (int i = 0; i < myarr.Length;i++){
result+=myarr[i];
}
string[] myarr = null;
char[] splitchar = { "<SP>" }; //ошибка - неявное преобразование
string sep = (string)splitchar; //ошибка - преобразование типов невозможно
myarr = richTextBox1.Text.Split(sep); //ошибка - метод имеет несколько недопустимых аргументов
for (int i = 0; i < myarr.Length;i++){
result+=myarr[i];
}
Код:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace SplitTest
{
class Program
{
static void Main(string[] args)
{
string words = "Нашёл по ссылке выше пример со сплитом, переделал метод нажатия на кнопку, но" +
"\tтипы что-то не хотят преобразовываться:.";
string[] split = words.Split(new Char[] { ' ', ',', '.', ':', '\t' });
foreach (string s in split)
{
if (s.Trim() != "")
Console.WriteLine(s);
}
}
}
}
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace SplitTest
{
class Program
{
static void Main(string[] args)
{
string words = "Нашёл по ссылке выше пример со сплитом, переделал метод нажатия на кнопку, но" +
"\tтипы что-то не хотят преобразовываться:.";
string[] split = words.Split(new Char[] { ' ', ',', '.', ':', '\t' });
foreach (string s in split)
{
if (s.Trim() != "")
Console.WriteLine(s);
}
}
}
}
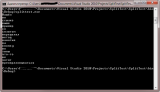
OR
Код:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace mySplitTest
{
public partial class Form1 : Form
{
string[] myarr = { };
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
myarr = richTextBox1.Text.Split(' ', ',', '.', ':', '\n');
richTextBox1.Clear();
for (int i = 0; i < myarr.Length; i++)
{
if (myarr[i] != "") richTextBox1.AppendText(myarr[i].Split(':', ';')[0] + "\n");
}
}
}
}
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace mySplitTest
{
public partial class Form1 : Form
{
string[] myarr = { };
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
myarr = richTextBox1.Text.Split(' ', ',', '.', ':', '\n');
richTextBox1.Clear();
for (int i = 0; i < myarr.Length; i++)
{
if (myarr[i] != "") richTextBox1.AppendText(myarr[i].Split(':', ';')[0] + "\n");
}
}
}
}
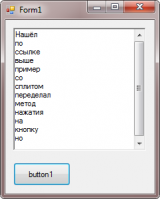
Цитата:
Так должно быть ?
Да, почти так.
Ох, и оказывается я правильно сначала писал, только сепаратор надо было в одинарные кавычки брать... Сейчас сделал, но работает только если в качестве сепаратора 1 символ. Если пишу несколько, то пишет ошибку "превышение допустимого числа символов в символьной константе". Я так понял, сепаратор должен быть типа char, но как преобразовать в char стороку из нескольких символов?
Цитата:
как преобразовать в char стороку из нескольких символов?
типа так
Код:
myarr = richTextBox1.Text.Split(new Char[] { ' ', ',', '.', ':', '\t' });
Код:
myarr = richTextBox1.Text.Split(new Char[]{'<SP>'});
от U+0000 до U+ffff
16-разрядный символ Юникода
Если ставлю, например '<' или ' ' или ':', то всё работает
Код:
string[] myarr = { };
string[] strSeparator = new string[] {"<SP>"};
....
....
....
myarr = richTextBox1.Text.Split(strSeparator, StringSplitOptions.None);
string[] strSeparator = new string[] {"<SP>"};
....
....
....
myarr = richTextBox1.Text.Split(strSeparator, StringSplitOptions.None);
Тегом <SP> в макросах пробел заменяется, и я планирую добавлять потом вместо него пробел через .join, но это не суть важно
Цитата:
myarr = richTextBox1.Text.Split(strSeparator, StringSplitOptions.None);
Во, теперь получилось! спс